2 Dimensional (2D).
By now, you should have an understanding how arrays work. The element number points to the data stored. I will briefly now explain 2D and 3D arrays. These are known as multidimensional array. You can have as many dimensional as you need, but make sure you have enough memory to store them.
float TempC[7][3];
This will look like a small spreadsheet. 2D arrays are reference in row and column format. So TempC will have 7 rows and 3 columns as illustrated in the diagram below.
I could also do this:-
float TempC[3][7];
Which would look like:-
You can use any method as long as you take care in storing and accessing the data. It is the norm to use the first method.
No special attention is needed to initialize the array. I would do the following for easier reading.
int table[4][3] = |
It just makes it easier to understand the way the data in the array is stored. It would not be wrong to do this:-
int table[4][3] = {1, 2, 3, 2, 4, 6, 3, 6, 9, 4, 8, 12};
if I wanted to print the array on lcd:-
Lesson 4.3
3D
Now I want to record temperature 3 times a day morning,
Looks confusing? Let me explain,
Each row from 0 to 11 is month which is [12];
Each alternate color column is day which is [31];
Each day column (0, 1 and 2) is time of day the temperature is recoded is [3].
To store / and retrieve data from 3D array:
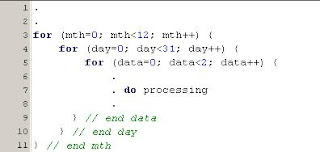
You can have as many dimensions that you need, but must take care of how much memory you have available. Example if you have an array of char sample[100][100][100], that is 1,000,000 bytes. Programming on a PC is OK but not for PIC.
Therein is a brief explanation on 3D arrays. If you do not understand, do not hesitate to leave comments us. Hope you have all understood the use of arrays. Next, I will explain a different kind of array in the next topic.
1 comment:
Lovely tutorial, extremely helpful for anyone learning multi-dimensional arrays.
Post a Comment